Phalcon – super simple REST API example
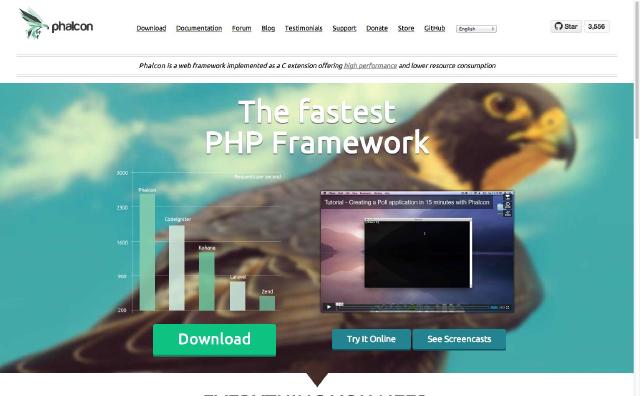
Throughout this first tutorial, i’ll walk you through the creation of an application with a simple REST API from the ground up. I assume you have Phalcon . installed already.
We start with basic setup described here: Micro – what we need it’s only couple lines of code:
$app = new Phalcon\Mvc\Micro();
$app->get('/say/welcome/{name}', function ($name) {
echo "Welcome $name!";
});
$app->handle();
Code is pretty clear – we are creating new instance of Micro Application, and capturing routes directed to ‘/say/welcome/…’ where after welcome you can pass some parameters.
What about proper responses ? What if we would like to spit out JSON or custom headers ?
we need Response object.
$response = new Phalcon\Http\Response();
$response->setStatusCode(200, "OK");
$response->setContent("Hello");
$response->send();
Let’s do this in proper way – using Dependency Management .
use Phalcon\DI\FactoryDefault,
Phalcon\Mvc\Micro,
Phalcon\Http\Response,
Phalcon\Http\Request;
$di = new FactoryDefault();
//Using an anonymous function, the instance will be lazy loaded
$di["response"] = function () {
return new Response();
};
$di["request"] = function() {
return new Request();
};
$app = new Micro();
$app->setDI($di);
$app->get('/api', function () use ($app) {
echo "Welcome";
});
$app->post('/api', function() use ($app) {
$post = $app->request->getPost();
print_r($post);
});
app->handle();
Easy – isn’t it ?
The last missing part of our puzzle is 404 handling. Because right now we can check out only one url /api.
Let’s add
$app->notFound(
function () use ($app) {
$app->response->setStatusCode(404, "Not Found")->sendHeaders();
echo 'This is crazy, but this page was not found!';
}
);
Just before $app->handle();
You can check this out here
next time – we are going to extend out app with models.