PHPUnit and CodeIgniter 3.0
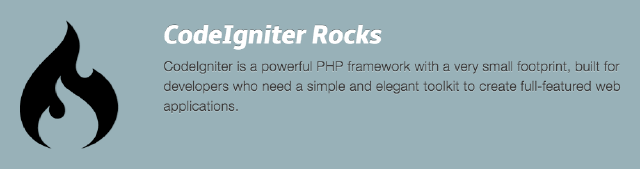
Quick tutorial how to setup proper unit testing with PHPUnit and CodeIgniter 3.0.
We need couple elements
- CodeIgniter – we are working with version 3.0rc3
- PHPUnit – latest one
If you don’t have phpunit installed globally, you can go with composer, just add section to your composer.json
{
"require-dev": {
"phpunit/phpunit": "4.1.*"
}
}
and thencomposer.phar install
and after while we have./vendor/bin/phpunit
working phpunit.
let’s create phpunit.xml.dist
next step will require create separate bootstrap file for PHPUnit. Just copy cp index.php tests/Bootstrap.php
We have to make 2 small amends.
- update
$system_path = '../system';
and$application_folder = '../application';
- change environment to testing
define('ENVIRONMENT', isset($_SERVER['CI_ENV']) ? $_SERVER['CI_ENV'] : 'testing');
CI is pretty bad with PHPUnit by default – so we need to modify one more element – output library.
Let’s enable hooks – $config['enable_hooks'] = TRUE;
in application/config/config.php create a hook in application/config/hooks.php
$hook['display_override'] = array(
'class' => 'DisplayHook',
'function' => 'captureOutput',
'filename' => 'DisplayHook.php',
'filepath' => 'hooks'
);
Actual hook code – application/hooks/DisplayHook.php
class DisplayHook
{
public function captureOutput()
{
$this->CI =& get_instance();
$output = $this->CI->output->get_output();
if (ENVIRONMENT != 'testing') {
echo $output;
}
}
}
And finally we can create out first test:
And we can see if it works:
./vendor/bin/phpunit
PHPUnit 4.1.6 by Sebastian Bergmann.
Configuration read from /Library/WebServer/Documents/coreadvisory/scraper/phpunit.xml.dist
.
Time: 117 ms, Memory: 5.00Mb
OK (1 test, 1 assertion)
Sources:
[taiar.github.io/php/2013/11/08/testing-codeigniter-applications-with-phpunit.html]( http://taiar.github.io/php/2013/11/08/testing-codeigniter-applications-with-phpunit.html